Python is smart--smart enough to know when code is attempting to assign the wrong value to an object. That’s when you might see the ValueError in Python. Throughout this blog post, we’ll go into detail about where this error sits in the Python Exception Class Hierarchy, what it looks like, how it differs from a TypeError, and how to fix it if you come across it.
Technical Rundown of the ValueError
All Python exceptions inherit from the BaseException class, as you’ll see below. Within the ValueError class, you’ll notice a few other exceptions, as well.
Here’s the breakdown:
- Base Exception
- Exception
- ValueError
- UnicodeError
- UnicodeDecodeError
- UnicodeEncodeError
- UnicodeTranslateError
- UnicodeError
- ValueError
- Exception
It’s a fairly straightforward hierarchy. Let’s continue on to what causes the Value Error in Python.
What Is A Python Value Error?
A ValueError in Python is a fairly simple concept. When you assign the wrong value to an object, you will get a ValueError.
Here’s what we mean by that. When you have the same type of object, but the incorrect value, Python won’t accept your code. It would be similar to trying to place a 65-inch TV into a 30-inch TV box. See the problem? The “value” of the TV won’t fit into the box. That is ValueError in a nutshell.

Make sure you don’t confuse a ValueError with a TypeError. Unlike a ValueError, a TypeError is raised when an operation is performed that is using an incorrect or unsupported object type. Using our TV example, it would be similar to taking a couch and trying to put it in a TV box. Python is smart enough to know that the two types don’t match.
While this is the most common reason for this error, you might see a ValueError pop-up for the following reasons:
- When you try to perform an operation when a value doesn’t exist
- Attempting to “unpack” more values than you have
- The value is impossible (For example, finding the root of a negative number will result in a ValueError exception)
Fortunately, there is a method you can use to find a ValueError Exception. If you have a ValueError, follow the steps listed below.
How to Handle a ValueError Exception
The best way to find and handle a ValueError Exception is with a try-except block. Place your statement between the try and except keywords. If an exception occurs during execution, the except clause you coded in will show up.
Here’s how it looks in action:


Now that you’ve set up a try-except block, it will be obvious where the exception is in your code. But is this the best way to find a ValueError? There’s an easier way to find Python exceptions in your code.
Why Use Airbrake
While the try-except method works to raise an error, it takes time. Imagine having to do this with every single type of exception. Airbrake Error Monitoring and Performance Monitoring makes it simple. When an error occurs within your Python application, you’ll have access to data that tells you what type of error it is, where it is, what environment it occurred in, occurrences, and other bits of aggregated data, making it incredibly easy to debug Python exceptions.
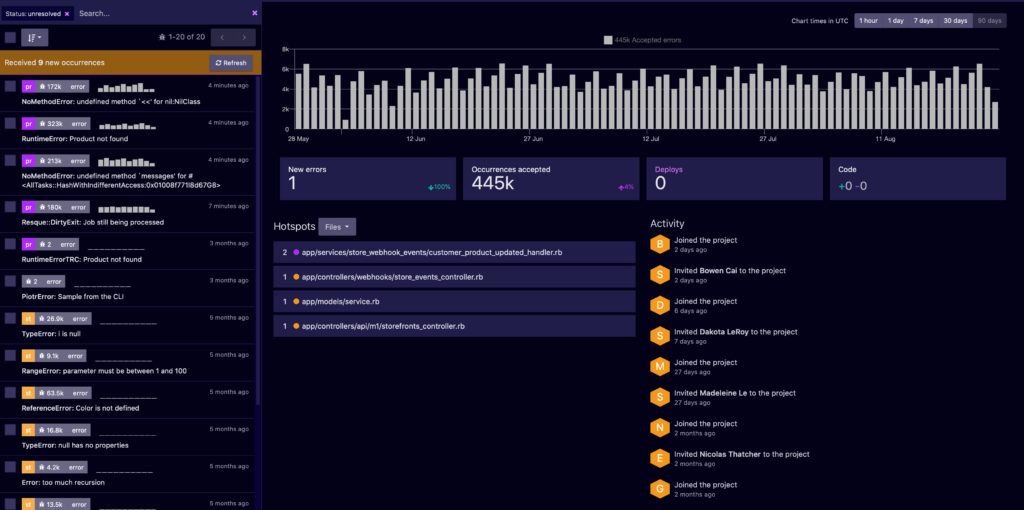
Not only does Airbrake provide incredibly minute details into errors, but it’s simple to install. See for yourself and head on over to the Python Error Monitoring page or check out the Airbrake-Python GitHub page.
Interested in trying Airbrake? Sign-up for our free 14-day trial.